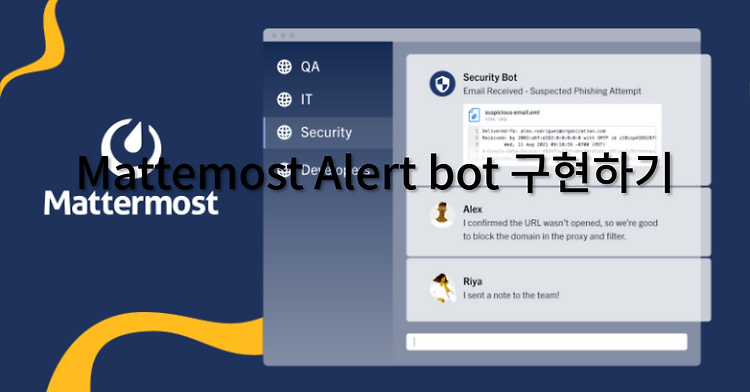
이전 포스팅
- 2024.01.31 - [분류 전체보기] - Mattermost alert bot 설계하기
- 2024.01.08 - [프로젝트/Github Rest API로 프로젝트 리뷰 대시보드 구성하기] - Grafana Dashboard에 Webhook 서버 (Mattermost 봇) 연결해서 알람 봇 만들기 - 작성중
- 2024.01.07 - [프로젝트/Github Rest API로 프로젝트 리뷰 대시보드 구성하기] - mattermost deploy 및 봇 사용해보기
필요 배경 지식
- Mattermost
- Typescript로 API 전송하기
Prerequisite
- Mattermost 로컬 실행
- Typescript API 서버 구성
Mattermost bot의 API 요청 기능을 어디에 붙일것인가.
기존의 API 서버에 그대로 붙여넣어도 될까에 대한 고민을 한번 해봤었다.
하지만, API 서버를 사용하는 시점과 bot이 API를 요청하여 update 시점이 crawler와 비슷하여 crawler의 코드 Workspace에 같이 구현하는것이 좋다고 생각했다.\
Github 리뷰 알람봇 UI 구성하기
그러면 bot의 내용 format은 어떻게 되어야할까 생각하다가 기능이 유사한 slack의 github plugin 포맷을 따라가보려고한다.
동일한 플러그인은 아니지만 직관적이기도하고 지라를 쓰는 사람도 납득하기 쉬우니 차용하면 좋을것 같았다.
이런 형태로 구현이 되어있다.
위와 같이 구현하기 위해서 mattermost documentation을 좀 보고 구현해보려고 한다.
Message attachments
Edit on GitHub Use message attachments For additional formatting options, and for compatibility with Slack non-markdown integrations, an attachments array can be sent by integrations and rendered by Mattermost. You can also add interactive message buttons
developers.mattermost.com
깃허브의 기능들을 보면서 approved나 commented 여부또한 alarm bot을 통해서 알려주면 좋긴하겠다는 생각이 들었다.
이 기능에 대해서도 추후 추가로 고민을 해보면 좋을것 같다.
일단은 기본적으로 노렸던 기능에 대해서 먼저 구현을 진행해보자
디자인은 아래와 같은 느낌으로 구성을 하려고 했다.
이를 위해서 mattermost API 에 아래와 같이 데이터를 포함하려고 구성했다.
const data = {
"channel_id": "9dynw6k8spn1trbd1s3nmokeke",
"props": {
"attachments": [
{
"fallback": "test",
"color": "#fff200",
"pretext": "Review requested from your co-workers",
"text": "including pr comment",
"title": "pr title",
"title_link": "pr-link",
"fields": [
{
"short": false,
"title": "Requested Reviewers",
"value": "add reviewers here"
},
],
"footer": "owner/reponame 1월 31일", // add owner/repo name here
"footer_icon": "https://github.githubassets.com/assets/GitHub-Mark-ea2971cee799.png" // Add github icon here
}
]
}
};
그럼 이제 원하는 데이터를 위의 포맷에다가 넣어주기만 하면 알람 내용은 구성이 될것 같고
알람을 보내는 시점을 처리하는 코드를 추가하면 대략적으로 알람봇의 역할은 가능할것으로 생각된다.
실제 코드 및 데이터를 추가하도록 아래와 같이 코드를 수정했고 하나의 예시는 아래와 같다.
export const sendPrAlarmByChannelId = async (channelId:string, Pr:Prs) => {
const data = {
"channel_id": channelId,
"props": {
"attachments": [
{
"fallback": "test",
"color": "#fff200",
"pretext": "Review requested from your co-workers",
"text": "Development team has requested your review for the following PR.",
"title": Pr.pr_name,
"title_link": Pr.html_url,
"fields": [
{
"short": false,
"title": "Requested Reviewers",
"value": Pr.requested_reviewers.toString(),
},
],
"footer": Pr.repo_id + " is repoid and " + Pr.created_at.getFullYear() + "년 " + Pr.created_at.getMonth() + "월 " + Pr.created_at.getDay() + "일 ", // add owner/repo name here
"footer_icon": "https://github.githubassets.com/assets/GitHub-Mark-ea2971cee799.png" // Add github icon here
}
]
}
};
...
이하 생략
}
특정 유저에게 bot이 direct message를 전달하는 방법
Mattermost API Reference
api.mattermost.com
Mattermost API 서버에서 확인하고 싶었다.
확인 결과 아래와 같은 과정을 통해서 direct message를 전달할 수 있는듯 보였다.
아래는 Direct Channel 을 생성하기 위한 API 링크이다.
Mattermost API Reference
api.mattermost.com
Create Direct Channel API를 통해서 direct message channel을 생성한 후 해당 Channel ID를 통해서 메세지를 보내는 것이다.
Direct Message Channel을 만들어 보자
위의 API와 함께 Header에 아래와 같이 user id와 bot id를 array로 넣어주면 그 두 대상에 대한 direct channel을 만들어 준다.
[
"bot-id",
"target-user-id"
]
실제 Typescript 함수를 아래와 같이 작성할 수 있겠다.
export const createDirectChannel = async (userId: string, otherUserId: string) => {
const data =
[
userId,
otherUserId
]
const config = {
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <bot-token>' // bot token
}
};
try {
const response = await axios.post('http://localhost:8065/api/v4/channels/direct', data, config);
return response.data.id
} catch (error) {
console.error(error);
}
}
문제는 mattermost의 admin이 아니거나 mattermost public API가 사용이 불가능하다면 userId와 botId를 확인하기가 아주 수월하지는 않다는 점이 있다.
일단 이 부분은 나중에 확인 가능하니 여기까지만 구현을 완료 해 두고 Channel에 대해서 알림을 줄 수 있는 기능만 추가해보도록 하면 좋을것 같다.
추가로 설계 및 구현 필요사항.
아래와 같은 로직이 추가로 필요할것이다.
1. 특정 유저가 특정 repo에 대한 알림을 enable/disable 하는 기능
- 위 기능을 지원하기 위한 DB 데이터가 필요함.
2. 특정 Channel이 특정 repo에 대한 알림을 enable/disable 하는 기능
- 이 또한 위 기능을 지원하기 위한 DB 데이터가 필요할듯 보인다.
'프로젝트 > Github Rest API로 프로젝트 리뷰 대시보드 구성하기' 카테고리의 다른 글
Github 변경에 대한 mattermost bot 코드를 Cursor AI 활용해서 재작성하기 (0) | 2025.02.09 |
---|---|
Mattermost alert bot 설계하기 (1) | 2024.01.31 |
Grafana Dashboard에 Webhook 서버 (Mattermost 봇) 연결해서 알람 봇 만들기 - 작성중 (0) | 2024.01.08 |
mattermost deploy 및 봇 사용해보기 (1) | 2024.01.07 |
NodeJS Express를 통해서 API 서버 구축 및 DB 업데이트 하기 (0) | 2023.12.25 |
개발 및 IT 관련 포스팅을 작성 하는 블로그입니다.
IT 기술 및 개인 개발에 대한 내용을 작성하는 블로그입니다. 많은 분들과 소통하며 의견을 나누고 싶습니다.